The Molecular System
Opkting’s molecular system, at its core, is a list of intramolecular fragments frag.Frag and intermolecular fragments dimerfrag.DimerFrag. The fragments in turn are lists of masses, atomic numbers and coordinates (cartesian or internal) along with a numpy array for the cartesian geometry. NIST values for masses, and atomic numbers can be easily retrieved through qcelemental e.g. qcelemental.periodictable.to_Z(‘O’)
A fragment for water:
>>> import optking
>>> zs = [1, 8, 1]
>>> masses = [1.007825032230, 15.994914619570, 1.007825032230]
>>> geometry = [-0.028413670411, 0.928922556351, 0.000000000000],
[-0.053670056908, -0.039737675589, 0.000000000000],
[ 0.880196420813, -0.298256807934, 0.000000000000]]
>>> fragment = optking.Frag(zs, geometry, masses)
The optimization coordinates can be added manually.:
>>> intcos = [optking.Stre(1, 2), optking.Stre(2, 3), optking.Bend(1, 2, 3)]
>>> fragment.intcos = intcos # intcos can also be added at instantiation
More typically, the coordinate system is automatically generated once the full molecular system is built and then edited if nessecary.:
>>> molsys = optking.Molsys([fragment])
>>> optking.make_internal_coords(molsys)
To control the automatic generation of coordinates the following keywords can be modified. For more information see the keyword documentation.
# TODO keyword documentation needs work (mostly copying pasting?)
optking.molsys Module
Classes
|
|
|
|
|
Return successive r-length combinations of elements in the iterable. |
|
Return successive r-length permutations of elements in the iterable. |
Class Inheritance Diagram

optking.frag Module
Classes
|
|
|
|
|
Return successive r-length combinations of elements in the iterable. |
Class Inheritance Diagram

optking.dimerfrag Module
Functions
|
Return the arc cosine (measured in radians) of x. |
|
Return the absolute value of the float x. |
|
Formats a Matrix for Logging or Printing |
|
Test the orient_fragment function to see if pre-determined target # coordinate values can be met. |
Classes
|
|
|
Set of (up to 6) coordinates between two distinct fragments. The fragments 'A' and 'B' have up to 3 reference atoms each (dA[3] and dB[3]). The reference atoms are defined in one of two ways: 1. If interfrag_mode == FIXED, then fixed, linear combinations of atoms in A and B are used. 2. (NOT YET IMPLEMENTED) If interfrag_mode == PRINCIPAL_AXES, then the references points are a. the center of mass b. a point a unit distance along the principal axis corresponding to the largest moment. c. a point a unit distance along the principal axis corresponding to the 2nd largest moment. # For simplicity, we sort the atoms in the reference point structure according to the assumed connectivity of the coordinates. ref_geom[0] = dA[2]; ref_geom[1] = dA[1]; ref_geom[2] = dA[0]; ref_geom[3] = dB[0]; ref_geom[4] = dB[1]; ref_geom[5] = dB[2]; # The six coordinates, if present, formed from the d{A-B}{0-2} sets are assumed to be the following in this canonical order: pos sym type atom-definition present, if --------------------------------------------------------------------------------- 0 RAB distance dA[0]-dB[0] always 1 theta_A angle dA[1]-dA[0]-dB[0] A has > 1 atom 2 theta_B angle dA[0]-dB[0]-dB[1] B has > 1 atom 3 tau dihedral dA[1]-dA[0]-dB[0]-dB[1] A and B have > 1 atom 4 phi_A dihedral dA[2]-dA[1]-dA[0]-dB[0] A has > 2 atoms and is not linear 5 phi_B dihedral dA[0]-dB[0]-dB[1]-dB[2] B has > 2 atoms and is not linear # :param A_idx: index of fragment in molecule list :type A_idx: int :param A_atoms: index of atoms used to define each reference point on A :type A_atoms: list of (up to 3) lists of ints :param B_idx: index of fragment in molecule list :type B_idx: int :param B_atoms: index of atoms used to define each reference point on B :type B_atoms: list of (up to 3) lists of ints :param A_weights (optional): weights of atoms used to define each reference point of A :type A_weights (optional): list of (up to 3) lists of floats :param B_weights (optional): weights of atoms used to define each reference point of B :type B_weights (optional): list of (up to 3) lists of floats :param A_lbl: name for fragment A :type A_lbl: string :param B_lbl: name for fragment B :type B_lbl: string :param The arguments are potentially confusing: :param so we'll do a lot of checking.:. |
|
|
|
Collection of weights for a single reference point. |
|
Class Inheritance Diagram
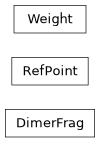